With Source.Python, the easiest way to accomplish this is to change their velocity directly with the teleport virtual function.
Syntax: Select all
entity.teleport(origin, angle, velocity)
Syntax: Select all
entity.physics_object.apply_force_center(velocity)

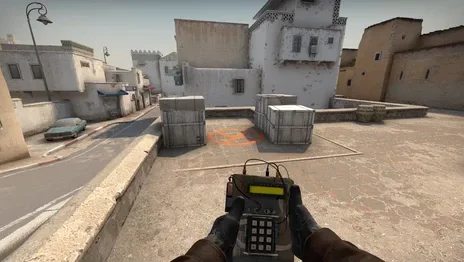
Here's a very simple class that you can use to make any entity bounce randomly with ease.
And here's how'd you use this to make entities bounce:
Syntax: Select all
from events import Event
@Event('bomb_dropped')
def bomb_dropped(event):
bouncy_bomb = bouncy_instances[event['entindex']]
# The bomb will only bounce when it's on the ground, until it is picked up.
bouncy_bomb.start_bouncing(tickrate=0.3, multiplier=1.3, ground_check=True)
Syntax: Select all
from entities.entity import BaseEntity
from entities.hooks import EntityPreHook, EntityCondition
@EntityPreHook(EntityCondition.is_player, 'drop_weapon')
def drop_weapon_pre(stack_data):
base_entity = BaseEntity._obj(stack_data[1])
bouncy_weapon = bouncy_instances[base_entity.index]
# The weapon will bounce once a second no matter where it is, either in the
# air or on the ground, for the next 10 seconds or until it is picked up.
bouncy_weapon.start_bouncing(
tickrate=1,
multiplier=0.5,
ground_check=False,
duration=10
)

By adjusting the bounce_multiplier, you can make entities wiggle, hit the skybox, and everything in between.
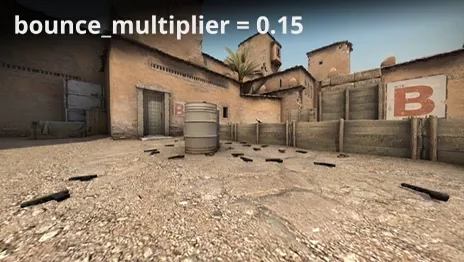
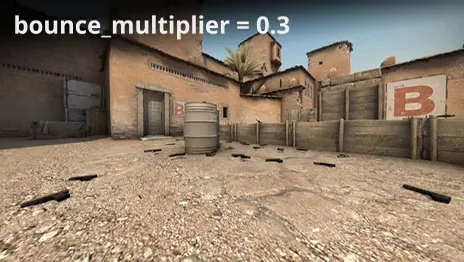
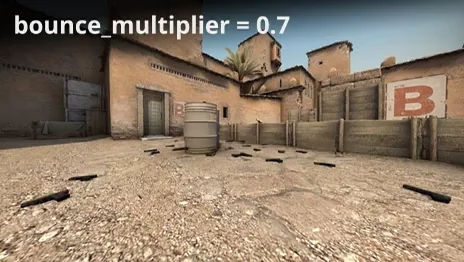
